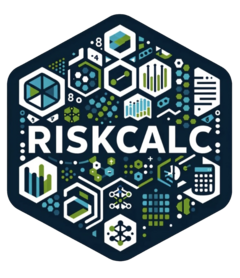
Build a risk calculator
risk_calculator.Rd
Create a (templated) risk calculator for www.riskcalc.org, optionally populating using an existing model object
Usage
risk_calculator(...)
# Default S3 method
risk_calculator(app_name = NULL, app_directory = NULL, ...)
# S3 method for class 'glm'
risk_calculator(
model,
app_name = NULL,
app_directory = NULL,
title = "",
citation = "",
label = "Predicted Value",
label_header = "Result",
value_header = "Value",
format = NULL,
labels = NULL,
levels = NULL,
placeholders = NULL,
...
)
# S3 method for class 'coxph'
risk_calculator(
model,
time,
app_name = NULL,
app_directory = NULL,
title = "",
citation = "",
label = "Survival Probability",
label_header = "Result",
value_header = "Value",
format = NULL,
labels = NULL,
levels = NULL,
placeholders = NULL,
...
)
Arguments
- ...
Generic arguments
- app_name
Application name (e.g.,
"AppExample"
for www.riskcalc.org/AppExample. If no name is provided, a generic name "riskcalc_app" is used.- app_directory
Location to export the application. If none provided, defaults to the working directory.
- model
- title
Title for risk calculator
- citation
Citation(s) and author information
- label
Label for the calculated value
- label_header
Header label for the result descriptor
- value_header
Header label for the result value
- format
Function to format the predicted value for display
- labels
Named character vector specifying labels for any inputs
- levels
Named list of named character vectors specifying labels for any factor levels
- placeholders
Named character vector specifying range limits for numeric input variables (of the form
c(var="<min>-<max>")
). Invokes calls tovalidate
enforcing the limits.- time
Single time point to calculate survival probability (see
summary.survfit
)
Value
A shiny
application
Examples
library(survival)
# Make a data set
dat <- gbsg
dat$meno <- factor(dat$meno)
# Build a model
mod <-
coxph(
formula = Surv(rfstime, status) ~ age + meno,
data = dat
)
# Time point of interest
time <- median(dat$rfstime) # 1084
# Create the risk calculator
if (FALSE) { # \dontrun{
risk_calculator(
model = mod,
time = time,
app_name = "BreastCancer",
app_directory = getwd(),
title = "Risk of Death or Recurrence in Breast Cancer",
citation =
paste0(
"Patrick Royston and Douglas Altman, External validation of a Cox prognostic",
"model: principles and methods. BMC Medical Research Methodology 2013, 13:33"
),
label_header = "`Probability Of Death or Recurrence`",
label = paste0("At time = ", time),
labels = c(age = "Age (years)", meno = "Menopausal Status"),
levels = list(meno = c(`0` = "Premenopausal", `1` = "Postmenopausal")),
placeholders = c(age = "21-80"),
format = function(x) paste0(round(100 * (1 - x), 2), "%")
)
} # }